How to generate Fluent Migrations and Update/Rollback from Visual Studio?
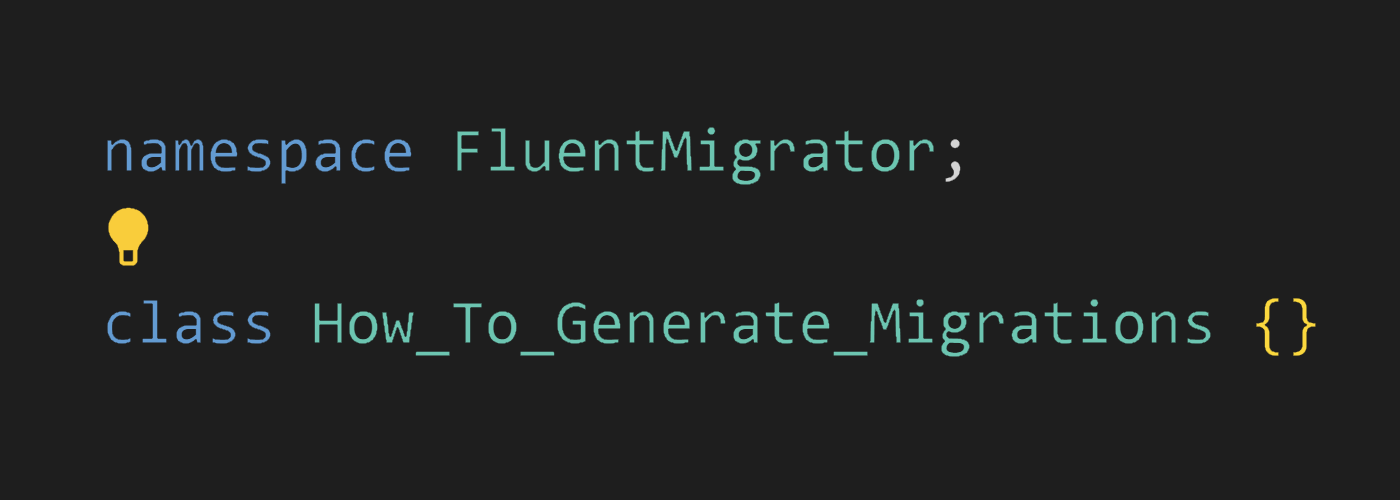
Discover how easily generate, update and rollback fluent migration in Visual Studio.
Problem
FluentMigrator is a great tool when you need to manage database changes. My recommendation is to check it if you didn’t try yet. But if you came from the Entity Framework world you will quickly find a downside.
EF Migrations allows you easily generate migrations by a command in the Power Manager Console
. You can generate a new migration, update, or rollback database. FluentMigrator at the same time does not have a migration generator and you should remember commands to apply/rollback migrations or you probably have a .bat file that does it for you.
So, what FluentMigrator is missing:
- migration generation with a timestamp key
- easy way to apply a migration
- easy way to rollback migration
IMHO it is a big disadvantage compared to EF Migrations because you need to spend more time and learn FluentMigrator commands and parameters.
Solution - Alt.FluentMigrator.VStudio
Alt.FluentMigrator.VStudio is a simple set of commands that solves all the listed problems above and even does more comparing to EF Migrations. It is developed as a NuGet package you can find here and also you can find quick documentation on GitHub: https://github.com/crimcol/Alt.FluentMigrator.VStudio.
1 Installation and configuration
Let’s say you develop a web application with .NET 6. and you have as a minimum two projects:
- WebApplication - ASP.NET Core app which contains a connection string;
- DbMigrations - class library responsible for database migrations.
1.1 Install FluentMigrator and FluentMigrator.Console packages
|
|
1.2 Install Alt.FluentMigrator.VStudio and a create configuration file
|
|
Once the package has been installed you can create a default migrations.json
configuration and set do not copy to output directory:
|
|
Let’s add a connection string to the WebApplication project if you don’t have it yet:
|
|
What we can change in the migrations.json
file
- ConnectionProjectName - the name of the project contains
Web.config
orApp.config
file with a connection string. - ConnectionName - connection name.
TestDb
in our case. - FluentMigrationToolPath - relative path to the console runner. Depends on what version of
FluentMigrator.Console
package you have might be located in another folder. - DbProvider - database provider. You can change provider here if you use MySql or PostgreSQL, etc. List of all providers here.
- MigrationFolder - folder name in your project where all migrations will be created. it also supports subfolders. ‘Migrations’ by default.
- ScriptsFolder - folder name for SQL scripts.
- TimeFormat - a time format is a number that will be used as a migration version and part of the file name. The time format “yyMMddHHmm” is also applicable and contains fewer characters.
"yyyyMMddHHmmss"
. In this case, you can avoid situations when another developer may generate a migration with the same version.2 Create a migration
To create a new migration you just need to run the command in the Package Manager Console:
|
|
20221128002948_InitialMigration.cs
file will look like this:
|
|
3 Create a migration with a script
For those who would like to write SQL script instead of writing C# code, you can generate a migration with a script.
|
|
As you can see this command will generate Up and Down SQL scripts and set the file path in fluent migration .cs file which I found extremely convenient.
4 Update database
Updating the database is the same simple as generating a new migration, just run the command Update-FluentDatabase
and it will apply all migrations which don’t exist in the database.
5 Rollback migration
|
|
What .Net Framework can be used?
In the GitHub repository you may find an Example that contains the following projects:
- Console app with .NET 6
- Web app with .NET 6
- Class Library with .NET 3.1
- Class Library with .NET Framework 4.8
I personally use it on a legacy project with .NET Framework 4.8 and on a new project with .NET 6.
Conclusion
Alt.FluentMigrator.VStudio NuGet package is a great helper to everyday work with database changes. If your application depends on a database and you often need to do database changes this small package is a huge time saver and for a whole team. You can simply generate a new migration, update, or rollback similar to Entity Framework.
I hope you enjoyed the demonstration and learned something new.
P.S. This package was developed by me. You can give a star to the GitHub repository and share it with your colleagues.
Resources
- FluentMigrator GitHub page: https://github.com/schambers/fluentmigrator
- Alt.FluentMigrator.VStudio GitHub page: https://github.com/crimcol/Alt.FluentMigrator.VStudio
- Checkout sample project on the GitHub repository